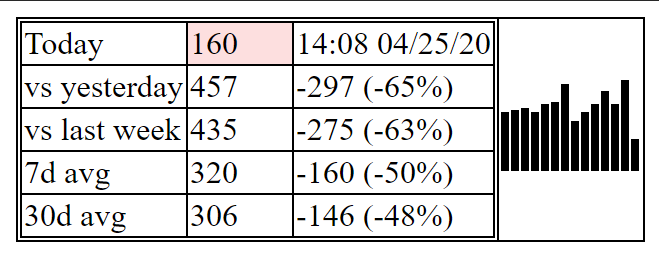
I like being able to keep an eye on everything that’s going on with the stuff I run. To that end I run a LibreNMS deployment in my house that monitors all my equipment, and I’v got a dashboard that gives me the vital statistics for everything I care about in one glance. One thing that has been sorely missing was a widget where I could see the stats for my WordPress sites, and I think I finally figured that out this week.
The biggest issue I was having was that WordPress’ API wants to use OAuth to generate the API token that you then use to get your stats. That’s a lot of work, but I found two shortcuts, one that worked better than the other.
stats_get_csv() via wp_load()
The first way to get your stats without needing to do a whole lot of work is to leverage your existing site’s key. If you have your site connected to WordPRess through Jetpack, then there’s a function you can leverage to extract the necessary information called stats_get_csv . This handy little guy will do all the hard work for you, querying the endpoint and returning the proper CSV of your stats.
There’s no need to go trawling through the code to find it either — if you include wp_load.php from your site’s root directory that will give you easy access to all the functions within WordPress including this one. Here’s a quick snippet of how you can use that config:
<?php require_once('wp-load.php'); $today=date('Y-m-d'); if( function_exists( 'stats_get_csv' ) ) { $return = stats_get_csv( 'views', 'days=1&limit=-1&end=' . $today ); } print_r($return); ?>
From there it should be a pretty quick process of converting that CSV output to an array and making up whatever visualization you prefer. There’s all kinds of information you can grab, and some pretty okay documentation of what you get.
While that does give you most of the information you need, there’s also a small drawback. Every time you hit that page it loads all of WordPress’ functions, including the logic that adds pageviews to your stats. So if you sit there and refresh it all day, especially if you’re not logged in as an admin, you’re going to be artificially inflating your stats.
The other issue is that you need to host that script on the WordPress site itself, to take advantage of the auth for the API key and being able to include that file. If you’re looking to keep this as secure as possible, or even just host this somewhere else like an internal server, then you’re going to want to query the API directly.
Querying stats.wordpress.com
WordPress had used an older API method to get stats in and out of their systems from remotely hosted deployments before moving to the JetPack plugin. These other methods relied solely on the use of an API key (that was conveniently emailed to you when you initially registered your WordPress account), and returned raw comma delineated results when queried. Much easier to work with than trying to implement OAuth for a simple little widget.
What this means is that not only do the calls to the API not count towards your stats, bbut they can be called independently of your WordPress site. For me, this meant that I was able to spin up a small docker container with just a web server and this PHP script and call it locally within my home network.
The query syntax is pretty simple, and you can make it all happen without mucking about in host headers. file_get_contents() and a concatenated URL string is all you need. Here’s an example of what that looks like:
https://stats.wordpress.com/csv.php?api_key=123456789abc&blog_id=155&table=referrers&days=30&limit=-1&summarize
That API key is invalid, so that link should actually take you to the documentation for the options available from that endpoint. There’s significantly more that seems to be exposed there, which is super useful.
Using this API I was able to slap together a simple PHP script that would query your site’s stats from anywhere and display them in a format that was useful to me. I’ve got all the code in this git repository, which is available to browse and download:
If you’re looking to deploy your own version of this script there should be all the information you need to get started on the readme file in that repository. Once deployed and integrated into my LibreNMS dashboard, here’s how it looks to me:
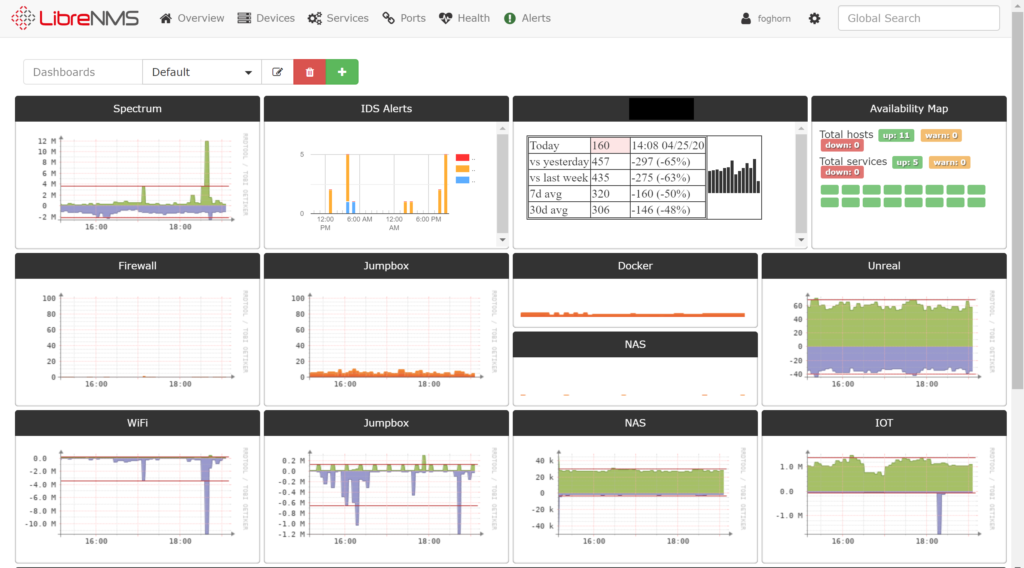
Super useful, in my opinion.